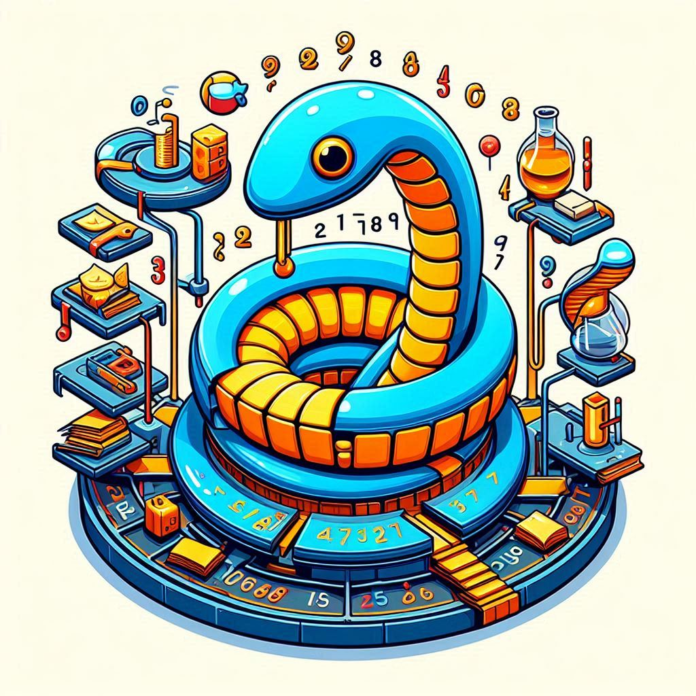
Are you trying to understand how to implement a factorial program in Python? You’re in the right place! In this article, we’ll explore the concept of factorials, how to write Python programs for calculating factorials, and why this concept is useful in programming. Let’s dive into the details and learn how to create a factorial program in Python with easy-to-follow steps.
Post Contents
What is a Factorial?
The factorial of a non-negative integer n
is the product of all positive integers less than or equal to n
. It is denoted as n!
. Factorials are commonly used in combinations, permutations, and other mathematical computations.
Mathematical Definition:
n!=n×(n−1)×(n−2)×…×1
Example:
5! = 5 × 4 × 3 × 2 × 1 = 120
For n = 0
, by definition, 0! = 1
.
How to Write a Factorial Program in Python?
In Python, there are multiple ways to calculate the factorial of a number. We’ll explore three approaches:
- Using a for loop
- Using recursion
- Using Python’s built-in function
1. Factorial Program in Python Using a For Loop
The for loop method is one of the simplest ways to calculate factorials.
# Factorial Program in Python using for loop
def factorial(n):
result = 1
if n < 0:
return "Factorial does not exist for negative numbers"
elif n == 0:
return 1
else:
for i in range(1, n + 1):
result *= i
return result
# Test the function
num = 5
print(f"The factorial of {num} is: {factorial(num)}")
Explanation:
- We initialize the result as 1.
- A loop runs from 1 to
n
(inclusive), multiplying each value with the result. - This method iterates through every number, multiplying them together to find the factorial.
2. Factorial Program in Python Using Recursion
Recursion is a technique where a function calls itself to solve smaller instances of the problem.
# Factorial Program in Python using recursion
def factorial_recursive(n):
if n < 0:
return "Factorial does not exist for negative numbers"
elif n == 0:
return 1
else:
return n * factorial_recursive(n - 1)
# Test the function
num = 5
print(f"The factorial of {num} is: {factorial_recursive(num)}")
Explanation:
- The base case is when
n = 0
, returning 1. - For all other numbers, the function calls itself with
n-1
, multiplyingn
by the result of the recursive call.
3. Factorial Program in Python Using Built-in Function
Python’s math
module provides a built-in function for calculating factorials, making it simple and efficient.
# Factorial Program in Python using built-in math module
import math
# Test the function
num = 5
print(f"The factorial of {num} is: {math.factorial(num)}")
Explanation:
- The
math.factorial()
function calculates the factorial directly, saving you the effort of writing the logic yourself.
Factorial Program Output
For all the above methods, if you input 5
, the output will be:
The factorial of 5 is: 120
Why is Factorial Important in Programming?
Factorials are crucial in solving various computational problems like:
- Combinatorics: Factorials help in calculating permutations and combinations.
- Probability Theory: Factorial calculations are often used in probability problems.
- Algorithm Complexity: Factorials appear in recursive algorithms and complex calculations.
Frequently Asked Questions (FAQs)
1. Can I calculate the factorial of a negative number?
No, the factorial is defined only for non-negative integers.
2. Is recursion or iteration better for factorial calculation?
Both approaches work well, but iteration (for loop) is generally more efficient for large numbers because recursion can cause a stack overflow.
3. Does Python have a built-in factorial function?
Yes, Python’s math
module has a built-in factorial()
function, which is optimized for factorial calculations.
Conclusion
We have explored how to write a factorial program in Python using three different methods: for loops, recursion, and Python’s built-in function. Whether you’re a beginner or an experienced coder, understanding these approaches will enhance your problem-solving skills in Python. Try implementing these methods in your next project and see the difference!
If you found this article helpful, share it with your fellow programmers and keep practicing your Python skills!