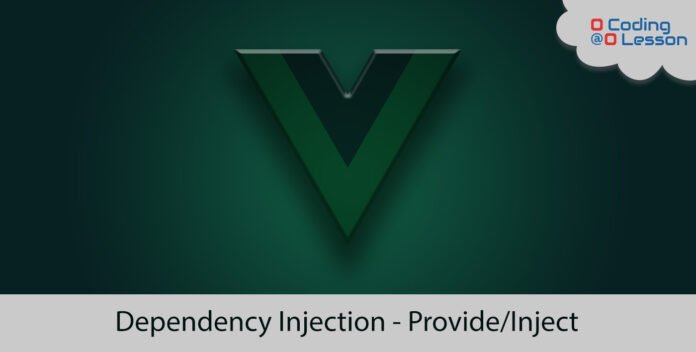
Mostly, props are used to pass the data from parent to child. In fact, it’s a good way to transfer data between simple parent-child components. But what if you want to transfer data from the parent component to all of their children’s components? In these tutorial, you’ll learn dependency injection with provide and inject.
Provide and Inject are introduced in Vue js >=2.2. Using provide in an ancestor component will inject functionality to all descendants components, no matter how deeply it’s structured. The provide option should be an object or a function that returns an object. Moreover, you can use an eventbus with the Vue JS instance in the provide option. But, It’s another topic, we will stick to using an object or function to provide option. In short, we will discuss it in another tutorial.
Most importantly, keep in mind that the variables passed from ancestor component to descendant component are not reactive. You can learn it from here.
Post Contents
# Prerequisite
Before starting, I assumed that you installed the Vue application with Vue CLI. However, you can install it using following commands. It will start your development server at localhost:8080.
npm install -g @vue/cli
vue create <folder-name>
cd <folder-name>
npm install && npm run serve
Then, open public > index.html and add bootstrap CDN into the head section.
/* public > index.html */
<head>
...
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.0-beta1/dist/css/bootstrap.min.css" rel="stylesheet"
integrity="sha384-giJF6kkoqNQ00vy+HMDP7azOuL0xtbfIcaT9wjKHr8RbDVddVHyTfAAsrekwKmP1" crossorigin="anonymous">
</head>
# Creating Components
Next, open src > App.vue and replace the following code. It contains provide option that is similar to data property and Home and About pages as well. As you can see in App.vue file Home and About pages are descendants components. Passing data with provide option will automatically be received in descendants components with inject option. Let’s create both pages too.
Now, check the message property provided in App.vue file in provide option. Also, check inject option used in Home or About page. You can use any function or object inside provide option.
/* src > App.vue */
<template>
<div id="app">
<div class="container my-3">
<Home></Home>
<About></About>
</div>
</div>
</template>
<script>
import Home from "@/views/Home";
import About from "@/views/About";
export default {
components: {
Home, About
},
provide() {
return {
message: "This is from provide option.",
}
}
}
</script>
/* src > views > Home.vue */
<template>
<div class="home">
<div class="alert alert-info">
Home: {{ message }}
</div>
</div>
</template>
<script>
export default {
name: 'Home',
inject: ['message']
}
</script>
/* src > views > About.vue */
<template>
<div class="about">
<div class="alert alert-warning">
About: {{ message }}
</div>
</div>
</template>
<script>
export default {
name: 'Home',
inject: ['message']
}
</script>
This is how you can use dependency injection with provide and inject in Vue JS. Thankyou for reading this tutorial.