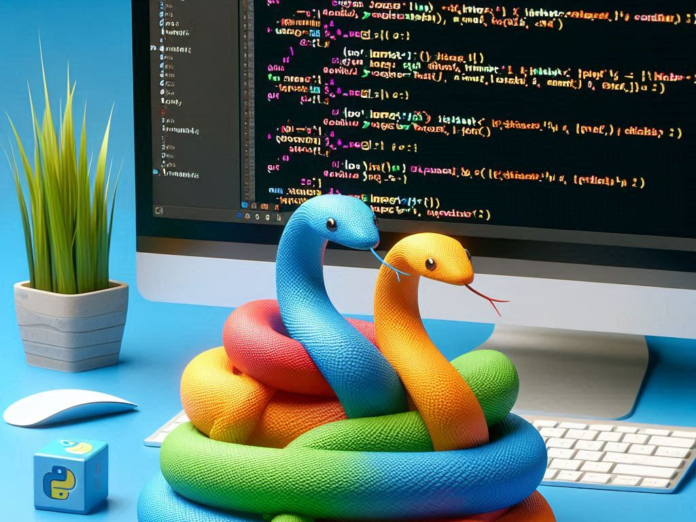
Control structures in python are an essential part of any programming language. In Python, control structures help you control the flow of your code, allowing you to execute code conditionally, repeat code, or choose between different outcomes. In this tutorial, we will explore the three main types of control structures in Python:
- Conditional Statements
- Loops
- Control Flow Modifiers
Let’s dive into each of them!
Post Contents
1. Conditional Statements
Conditional statements in Python let you make decisions in your code based on conditions. The most commonly used conditional statement is the if
statement.
1.1 The if
Statement
The if
statement allows you to execute a code block only if a specified condition is true.
Syntax:
if condition:
# code to execute if condition is true
Example:
x = 10
if x > 5:
print("x is greater than 5")
Output:
x is greater than 5
1.2 The else
Statement
The else
statement provides an alternative block of code that runs if the condition in the if
the statement is false.
Syntax:
if condition:
# code to execute if condition is true
else:
# code to execute if condition is false
Example:
x = 3
if x > 5:
print("x is greater than 5")
else:
print("x is less than or equal to 5")
Output:
x is less than or equal to 5
1.3 The elif
Statement
The elif
(short for else if) statement allows you to check multiple conditions before reaching the else
block.
Syntax:
if condition1:
# code if condition1 is true
elif condition2:
# code if condition2 is true
else:
# code if none of the above conditions are true
Example:
x = 7
if x > 10:
print("x is greater than 10")
elif x > 5:
print("x is greater than 5 but less than or equal to 10")
else:
print("x is less than or equal to 5")
Output:
x is greater than 5 but less than or equal to 10
2. Loops
Loops allow you to repeat a block of code multiple times. Python supports two types of loops:
for
loopswhile
loops
2.1 The for
loop
The for
loop in Python is used to iterate over a sequence (like a list, tuple, dictionary, set, or string).
Syntax:
for item in sequence:
# code to execute for each item in the sequence
Example:
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
Output:
apple
banana
cherry
2.2 The while
Loop
The while
loop repeats a code block as long as a specified condition is true.
Syntax:
while condition:
# code to execute while condition is true
Example:
x = 0
while x < 5:
print(x)
x += 1
Output:
0
1
2
3
4
3. Control Flow Modifiers
Python provides several keywords to alter the normal flow of loops or conditionals.
3.1 The break
Statement
The break
statement is used to exit a loop before it has gone through all iterations.
Example:
for i in range(10):
if i == 5:
break
print(i)
Output:
0
1
2
3
4
3.2 The continue
Statement
The continue
statement is used to skip the current iteration of a loop and continue with the next iteration.
Example:
for i in range(5):
if i == 3:
continue
print(i)
Output:
0
1
2
4
3.3 The pass
Statement
The pass
statement does nothing. It can be used when a statement is syntactically required, but you don’t want to execute any code.
Example:
for i in range(5):
if i == 3:
pass # do nothing
print(i)
Output:
0
1
2
3
4
Conclusion
Control structures in Python allow you to control the flow of your program, making it more dynamic and interactive. Whether you are making decisions with if-else
statements, repeating code with loops, or modifying the flow using break
, continue
, or pass
, mastering control structures is crucial for becoming proficient in Python programming.
Understanding how and when to use each control structure will help you write more efficient and readable code. Happy coding!