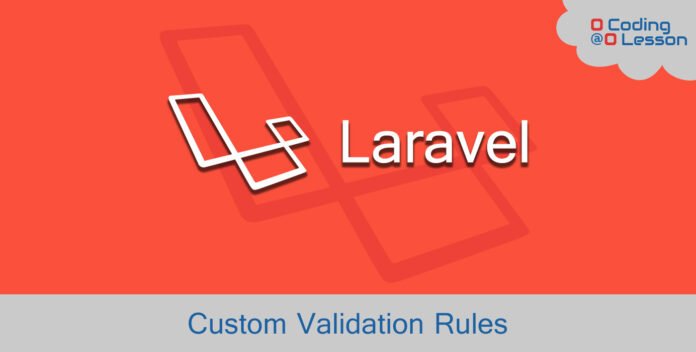
In this chapter, you are going to learn how to create custom validation rules in laravel. Although, Laravel provides lots of validations rules. But this method will help you to create your own validation rule. I’m using laravel 7 for this tutorial. So, let’s get started.
I believed that you installed laravel and you have basic knowledge about validation. To check available validation rules click here.
I’m simply using the phone number field in the form to validate with custom validation rules. To do that, create a simple form with the following code into welcome.blade.php.
/* resources > views > welcome.blade.php */
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Laravel Custom Rules</title>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.4.1/css/bootstrap.min.css">
</head>
<body>
<div class="container mt-5">
<form action="{{ route('form.store') }}" method="post">
@csrf
<div class="form-group">
<label>Phone Number</label>
<input type="text" name="phone" class="form-control">
@if($errors->has('phone'))
<span class="text-danger">{{ $errors->first('phone') }}</span>
@endif
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
</div>
</body>
</html>
Now, let’s create FormController.php to submit the form.
php artisan make:controller FormController
Next, create index and store function in FormController.php
/* app > Http > Controllers > FormController.php */
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class FormController extends Controller
{
public function index()
{
return view('welcome');
}
public function store(Request $request)
{
}
}
After that, create necessary routes.
/* routes > web.php */
<?php
Route::get('/', 'FormController@index');
Route::post('form/store', 'FormController@store')->name('form.store');
Post Contents
# Creating Validation Rule
To generate a new validation rule laravel provides a make:rule artisan command. So, let’s create new PhoneValidation rule using this command. This will create the new rule in the app/Rules directory.
php artisan make:rule PhoneValidation
The newly created PhoneValidation rule includes two methods. The passes method accepts the attribute value and name, and it will return true or false to determine the attribute value is valid or not. The message method will return the validation error message. Now, modify the PhoneValidation file as below.
/* app > Rules > PhoneValidation.php */
<?php
namespace App\Rules;
use Illuminate\Contracts\Validation\Rule;
class PhoneValidation implements Rule
{
public function passes($attribute, $value)
{
return preg_match("/([+]?\d{1,2}[.-s]?)?(\d{3}[.-]?){2}\d{4}/", $value);
}
public function message()
{
return 'Please enter valid phone number.';
// OR return attribute name
// return 'Please enter valid :attribute.';
}
}
Once the rule has been set, you can attach it to the validation rules. Of course, validations can be used in controllers, but there are better ways to do that. Laravel provides form requests methods for separate validation logic from the controller’s business logic.
# Creating Form Request
Use following command to create form request, this will create a new request in app > Http > Requests folder. You can use any name for form request.
php artisan make:request FormValidationRequest
Open FormValidationRequest.php and update as below. You need to import PhoneValidation and use it into validation rules.
/* app > Http > Requests > FormValidationRequest.php */
<?php
namespace App\Http\Requests;
use App\Rules\PhoneValidation;
use Illuminate\Foundation\Http\FormRequest;
class FormValidationRequest extends FormRequest
{
public function authorize()
{
return true;
}
public function rules()
{
return [
'phone' => ['required', new PhoneValidation]
];
}
public function messages()
{
return [
'phone.required' => 'Please enter phone number.'
];
}
}
Finally, import FormValidationRequest into FormController and update store method as below.
/* app > Http > Controllers > FormController.php */
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Http\Requests\FormValidationRequest;
class FormController extends Controller
{
public function index()
{
return view('welcome');
}
public function store(FormValidationRequest $request)
{
return "Form submitted";
}
}
That’s it. Try to validate the form with phone number. PhoneValidation will throw an error as expected.