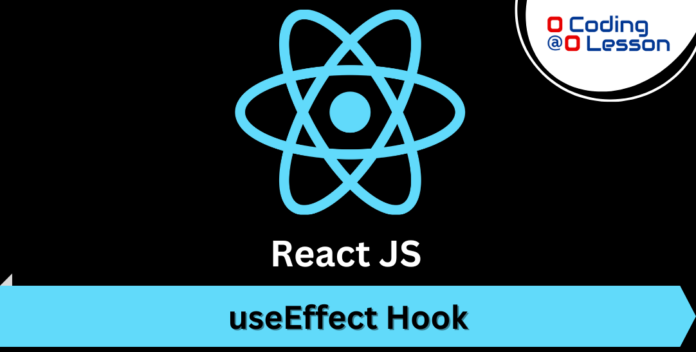
React is a popular JavaScript library for building user interfaces, and the useEffect hook is one of its key features. The useEffect hook allows you to synchronize a component with an external system, such as a web API or a real-time data stream. In this blog post, we will explore how to use the useEffect hook in a React application.
First, it’s important to understand the basic syntax of the useEffect hook. The hook takes two arguments: a callback function that contains your side effects, and a dependency array. The callback function is executed after every render, and the dependency array is used to determine when the hook should re-run.
Here’s an example of how you might use the useEffect hook to fetch data from an external API and update the component’s state:
import { useEffect, useState } from 'react';
function MyComponent() {
const [data, setData] = useState(null);
useEffect(() => {
fetch('https://my-api.com/data')
.then(response => response.json())
.then(data => setData(data));
}, []);
return data ? <div>{data.name}</div> : <div>Loading...</div>;
}
In this example, the useEffect hook is being used to fetch data from an external API and update the component’s state. The empty dependency array means that the hook will only run once, on the initial render.
You can also use the useEffect hook to handle cleanup logic. The useEffect hook returns a cleanup function that is called before the hook is executed again. This can be used to cancel any ongoing network requests, remove event listeners, or clean up any other resources.
import { useEffect, useState } from 'react';
function MyComponent() {
const [data, setData] = useState(null);
useEffect(() => {
const controller = new AbortController();
const { signal } = controller;
fetch('https://my-api.com/data', { signal })
.then(response => response.json())
.then(data => setData(data));
return () => controller.abort();
}, []);
return data ? <div>{data.name}</div> : <div>Loading...</div>;
}
In this example, the useEffect hook is being used to fetch data from an external API, and the cleanup function is used to cancel the ongoing network request if the component is unmounted or the hook is re-run.
The useEffect hook is a powerful tool that can help you synchronize your components with external systems and handle cleanup logic. By understanding the basic syntax and the role of the dependency array, you can use the hook to build more robust and performant React applications.
In summary, the useEffect hook allows you to synchronize a component with an external system and handle cleanup logic, by taking a callback function and a dependency array as arguments. It is a powerful tool for building more robust and performant React applications.