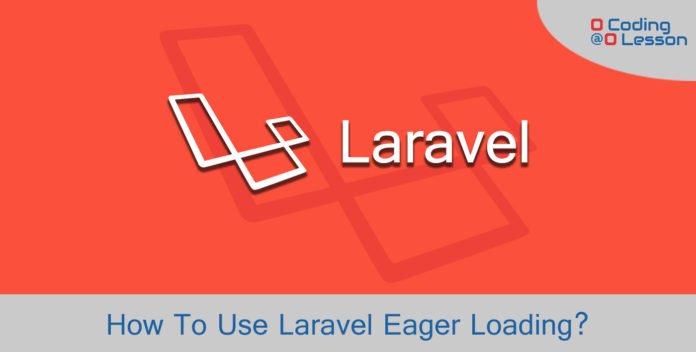
Using laravel eloquent relationships always resulted in “lazy loaded” by default. In this tutorial, you will learn how to use Laravel Eager Loading. Let’s Think of every employee has one job. To understand eager loading, you need Laravel One To One Relationship to connect both models. Please setup laravel project with an above link with laravel eloquent one to one relationship.
Post Contents
# Installing Laravel Debugbar
After setting up project install laravel debugging package with the following command. Using this package you will visually understand how eager loading works. You can skip installing this package, but its good practice to have this package in development mode in your all laravel project.
composer require barryvdh/laravel-debugbar --dev
Register the service into the app.php’s providers array.
/* config > app.php */
'providers' => [
Barryvdh\Debugbar\ServiceProvider::class,
]
Similarly, register the facade into aliases array.
/* config > app.php */
'aliases' => [
'Debugbar' => Barryvdh\Debugbar\Facade::class,
]
That’s It! You successfully configured laravel debugbar package. You can disable it by setting APP_DEBUG=false in to .env file. You will check used queries in query tab in debugbar.
# Understanding Eager Loading
Create TestController and put following logic into it. We are passing all employees in welcome page.
php artisan make:controller TestController
/* app > Http > Controllers > TestController.php */
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Employee;
class TestController extends Controller
{
public function index()
{
$employees = Employee::all();
return view('welcome', compact('employees'));
}
}
Replace route in web.php
/* routes > web.php */
<?php
Route::get('/', 'TestController@index');
Into welcome page put following code to loop through all employees
/* resources > views > welcome.blade.php */
@foreach($employees as $employee)
<p>Name: {{ $employee->name }}</p>
@endforeach
As you can see in debugbar laravel using one query to get all employees. Thats fine.
Now, find all employees company name from job table with one to one relationship. To do so update welcome page as below.
/* resources > views > welcome.blade.php */
@foreach($employees as $employee)
<p>Name: {{ $employee->name }} <br> Job: {{ $employee->job->name_of_company }}</p>
@endforeach
This is lazy loading. N + 1 queries executed for finding all employees and their jobs. Where N is a number of employees. We have 10 records that’s why it’s using 11 queries. Let’s think of 1000 employees, there will be major performance issues.
Now, comes the eager loading to play. Laravel eager loading using with method. Update TestController a little bit.
/* app > Http > Controllers > TestController.php */
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Employee;
class TestController extends Controller
{
public function index()
{
$employees = Employee::with('job')->get();
return view('welcome', compact('employees'));
}
}
Check welcome page. As you can see in debugbar, with eager loading you can get same result by using only 2 queries.