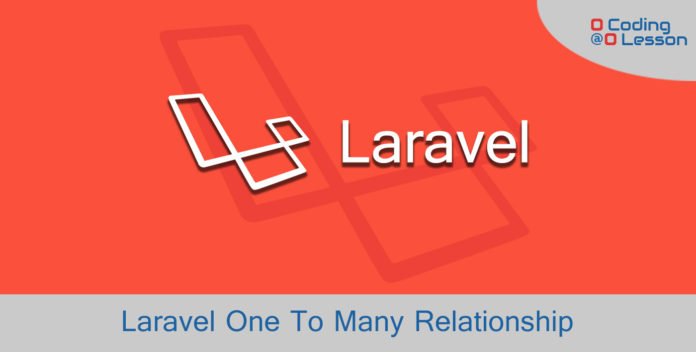
In this chapter, we will learn how to use Laravel Eloquent One To Many Relationship. You can connect a single modal to multiple amounts of the modal. Let’s take an example every article has many comments, a book has many chapters and a country has many states. We will use the country and states throughout this tutorial. So let’s get started.
Post Contents
# Requirements
- Laravel installed in your system. Click Here to know how to install Laravel Framework
- Laravel basic relationship understanding. Check Laravel One To One Relationship
# Create Models With Migrations
Go to PHP project directory and use the following command to install Country and State model and migrations.
php artisan make:model Country -m
php artisan make:model State -m
Using -m option will automatically create migration for model in database > migration folder.
In Country model and replace below code.
/* app > Country.php */
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class Country extends Model
{
protected $fillable = ["name"];
}
Likewise, In State model and replace below code.
/* app > State.php */
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class State extends Model
{
protected $fillable = ["name"];
}
# Create Database Schema In Migrations.
Define schema in countries migration.
/* database > migrations > *_create_countries_table.php */
public function up()
{
Schema::create('countries', function (Blueprint $table) {
$table->bigIncrements('id');
$table->string('name');
$table->timestamps();
});
}
Similarly, define schema in states migration.
/* database > migrations > *_create_states_table.php */
public function up()
{
Schema::create('states', function (Blueprint $table) {
$table->bigIncrements('id');
$table->unsignedBigInteger('country_id');
$table->string('name');
$table->timestamps();
$table->foreign('country_id')->references('id')->on('countries');
});
}
Now, migrate two tables with following command.
php artisan migrate
# Defining Relationship
Finally, we are ready to define laravel eloquent one to many relationship between Country and State models.
First of all, define states function (take any name you want) with hasMany method in Country Model. Every One To Many relation must have hasMany method connected to his related model.
/* app > Country.php */
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class Country extends Model
{
protected $fillable = ["name"];
public function states()
{
return $this->hasMany('App\State');
}
}
Similarly, in State model define country function (you can take any name you want) with belongsTo method in it. That is inverse relationship.
/* app > State.php */
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class State extends Model
{
protected $fillable = ["name"];
public function country()
{
return $this->belongsTo('App\Country');
}
}
Thats It! You created one to many relationship between Country and State model. You just need to remember hasMany and belongsTo functions defined in models.
# Usage
Suppose if you have country object and you want to find all states of the country then you can use hasMany function defined in Country model.
$country = App\Country::find(1);
$states = $country->states;
foreach($states as $state)
{
echo $state->name;
}
Similarly, If you want to find country from state object then you can use belongsTo function defined in State model.
$state = App\State::find(1);
$state->country->name;
Like!! Thank you for publishing this awesome article.
Exactly which is near to my heart… Take care! Where are your contact details though?
Saved as a favorite, I really like your web site!
Saved as a favorite, I like your web site! blog articles.