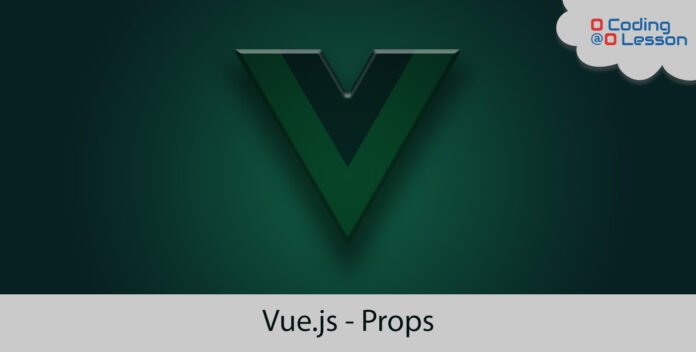
Basically, vuejs props are useful when passing data from an ancestor (parent) component to its descendant (child) component. To do that, pass an HTML attribute from the parent component. After that, you can access it with props property in the child component. As easy as that.
In this tutorial, you will learn how to use vuejs props. In the first place, I believe that you have basic knowledge of vuejs and its components. Alongside, you can read all stuff about props here.
Post Contents
# Using Props
At this moment, create two components App.vue and Home.vue. Now, let’s pass data from App.vue to Home.vue with using props. Keep in mind that HTML attributes are case-insensitive. Therefore, you can use kebab-case or camelCase when defining props. However, I’m using a simple way to pass message and visitor properties to the child component. After that, we’ll dig deeper.
/* src > App.vue */
<template>
<div id="app">
<Home message="This is homepage" visitor="5"></Home>
</div>
</template>
<script>
import Home from "@/Home";
export default {
components: {
Home
}
}
</script>
/* src > Home.vue */
<template>
<div class="home">
<p>{{ message }}</p>
<p>You have {{ visitor }} visitor</p>
</div>
</template>
<script>
export default {
name: 'Home',
props: ["message", "visitor"]
}
</script>
As you can see, in App.vue file message and visitor attributes passed when using Home.vue as a child component. As a result, you can receive both attributes in Home.vue file using props as shown.
Furthermore, dynamic properties also supported when using props.
/* src > App.vue */
<template>
<div id="app">
<Home :message="message" :visitor="visitor"></Home>
<button @click="visitor++">Increase Visitor</button>
</div>
</template>
<script>
import Home from "@/Home";
export default {
data() {
return {
message: "Dynamic Message",
visitor: 0
}
},
components: {
Home
}
}
</script>
# Types Of Props
As shown above, we’ve used props as an array with string values. On the other hand, you can use props with a specific type of values. In that case, you need to use props as an object. In object, keys are the name of props when the values are a type of the name. To do so, change in Home.vue.
/* src > Home.vue */
<template>
<div class="home">
<p>{{ message }}</p>
<p>You have {{ visitor }} visitor</p>
</div>
</template>
<script>
export default {
name: 'Home',
props: {
message: String,
visitor: Number
}
}
</script>
# Validating Props
Equally important, you can validate prop types too. In effect, vue will inform you through the javascript console. Validation with props requires an object. Let’s take a look.
/* src > Home.vue */
<template>
<div class="home">
<p>{{ message }}</p>
<p>You have {{ visitor }} visitor</p>
</div>
</template>
<script>
export default {
name: 'Home',
props: {
message: {
type: String,
require: true,
},
visitor: {
type: Number,
require: true
}
}
}
</script>
That’s all for the vuejs props. Please support with comment or sharing this article. Thankyou.