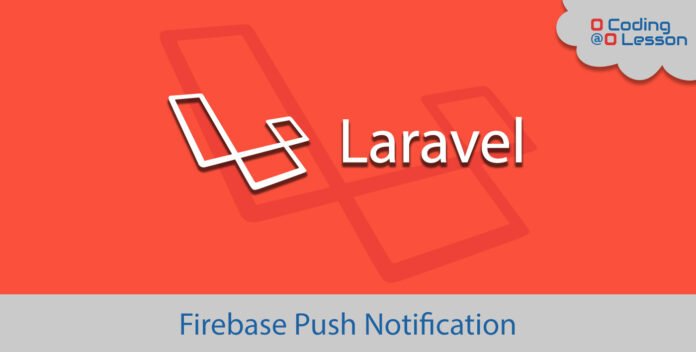
In this tutorial, we’ll learn how to send a firebase push notification using laravel. You might need to send push notifications to Android or IOS devices in some applications. We’ll use the FCM (Firebase Cloud Messaging) platform to send push notifications. FCM was previously called GCM (Google Cloud Messaging).
To explain, you can either use the laravel composer package or CURL request to send firebase push notifications. With this intention, we’re using brozot/laravel-fcm composer package and a CURL request for your information.
Post Contents
# Prerequisite
In the first place, you must be familiar with the laravel framework. During this tutorial, I’ll use Laravel 5.5. You can install laravel and it’s development server on localhost:8000 using the following commands.
composer create-project --prefer-dist laravel/laravel notification
cd notification
php artisan serve
Sooner or later, you need at least one fcm_token (or device_token) of your Android or IOS device. Along with that, you need an FCM server key for the authorization for the account. First of all, you need to create a project in firebase console. Now, enter the project and click on Project overview > Prject settings. Finally, clicking on the cloud messaging tab will give you a chance to create server key that known as fcm server key. Along with the Server Key, keep your Sender ID with you which is below the Serve Key. It will be useful when sending a notification with the composer package.
# Stop & Read This
At this instant, let’s know about some tips about notification payloads. Generally, notification and data payloads are available to send push notifications. Keep in mind, some rules must be followed before sending a notification. The first thing to remember, data payloads must be handled by Android or IOS developers. It will make a notification clickable and redirectable to any application page. On the other hand, using only notification payload will open the application with no specific redirection. As you can see below, single and multiple notifications will be sent using the following syntaxes:
<?php
/* To send notification for single fcm token */
$notificationData = [
'token' => 'your fcm token',
];
/* To send notification for multiple fcm token you need an array */
$notificationData = [
'registration_ids' => ['fcm token 1', 'fcm token 2', 'fcm token 3'],
];
# Notification Payload
Keep in mind, notification payload will be delivered to the tray when the app is in the background. Notification payload only supports the title, body, and image keys. For example, to send a payload notification to fcm_token you can use:
<?php
/* To send single notification with notification payload */
$notificationData = [
'token' => 'your fcm token',
'notification' => [
'title' => 'Your title here',
'body' => 'Your message here',
'image' => 'Your image link here',
]
];
/* To send multiple notification with notification payload */
$notificationData = [
'registration_ids' => ['fcm token 1', 'fcm token 2', 'fcm token 3'],
'notification' => [
'title' => 'Your title here',
'body' => 'Your message here',
'image' => 'Your image link here',
]
];
# Data Payload
On the other hand, in the data payload, you can use custom key-value pair to inject other data to send in the notification. Most importantly, data payload will use when the application is in the background. When a user clicks on the notification then data payload’s custom data will work. To point out, you can use both payloads at the same time.
<?php
/* To send single notification with data payload */
$notificationData = [
'token' => 'your fcm token',
'data' => [
'username' => 'Your username here',
'description' => 'Your message here',
'media' => 'Your image link here',
'category' => 'Your category name',
'link' => 'Category Link'
]
];
/* To send multiple notification with data payload */
$notificationData = [
'registration_ids' => ['fcm token 1', 'fcm token 2', 'fcm token 3'],
'data' => [
'username' => 'Your username here',
'description' => 'Your message here',
'media' => 'Your image link here',
'category' => 'Your category name',
'link' => 'Category Link'
]
];
# Sending Notification With CURL
With this in mind, let’s send a push notification using the CURL request. I’m using both payloads as discussed.
<?php
$notificationData = [
'registration_ids' => ['fcm token 1', 'fcm token 2', 'fcm token 3'],
'notification' => [
'title' => 'Your title here',
'body' => 'Your message here',
'image' => 'Your image link here',
]
'data' => [
'username' => 'Your username here',
'description' => 'Your message here',
'media' => 'Your image link here',
'category' => 'Your category name',
'link' => 'Category Link'
]
];
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, "https://fcm.googleapis.com/fcm/send");
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($notificationData));
curl_setopt($ch, CURLOPT_HTTPHEADER, array('Content-Type: application/json', 'Authorization:key=' . $fcm_server_key));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, false);
curl_exec($ch);
curl_close($ch);
?>
# Sending Notification With Composer Package
You can use choose a composer dependency instead of a CURL request to simplify and extend functionality. Laravel FCM is a great package available. This package is available with lots of functionality to send different types of notifications. For example, downstream messages, topic messages, group messages, notification messages, data messages.
First of all, let’s install that package with the following command.
composer require brozot/laravel-fcm
If you are using, laravel < 5.5 then you need to register the provider into the config > app.php file. To do that:
/* config > app.php */
<?php
/* Register the provider */
'providers' => [
LaravelFCM\FCMServiceProvider::class,
],
/* Add the facade alias */
'aliases' => [
'FCM' => LaravelFCM\Facades\FCM::class,
'FCMGroup' => LaravelFCM\Facades\FCMGroup::class,
],
This configuration is not necessary when you are using the laravel version above 5.5. By the way, you can publish the package config file with the following command.
php artisan vendor:publish --provider="LaravelFCM\FCMServiceProvider"
After publishing the configuration, open your .env file. Now place your Server Key and Sender Id as below. You can put it directly into config > fcm.php file too.
FCM_SERVER_KEY=your_server_key
FCM_SENDER_ID=your_sender_id
There are lots of ways to send a notification with this package. But I’m using a simple way. I’m using notification and data payload both.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use LaravelFCM\Facades\FCM;
use LaravelFCM\Message\OptionsBuilder;
use LaravelFCM\Message\PayloadDataBuilder;
use LaravelFCM\Message\PayloadNotificationBuilder;
class PushNotificationController extends Controller
{
public function send()
{
config(['fcm.http.server_key' => $shop->fcm_server_key]);
config(['fcm.http.sender_id' => $shop->fcm_sender_id]);
// To build options
$optionBuilder = new OptionsBuilder();
$optionBuilder->setTimeToLive(60 * 20);
$option = $optionBuilder->build();
// To build notification payload
$notificationBuilder = new PayloadNotificationBuilder($unSentScheduledNotification->message);
$notificationBuilder->setBody([
'title' => 'Your title here',
'body' => 'Your message here',
'image' => 'Your image link here',
]);
$notification = $notificationBuilder->build();
// To build data payload (Optional)
$dataBuilder = new PayloadDataBuilder();
$dataBuilder->addData([
'username' => 'Your username here',
'description' => 'Your message here',
'media' => 'Your image link here',
'category' => 'Your category name',
'link' => 'Category Link'
]);
$data = $dataBuilder->build();
// to send single notification
$token = 'your token here';
FCM::sendTo($token, $option, $notification, $data);
// to send multiple notifications
$tokens = ['fcm token 1', 'fcm token 2', 'fcm toke 3'];
FCM::sendTo($tokens, $option, $notification, $data);
}
}
So, that’s it for firebase push notification. I hope it helps a lot for sending push notifications. Thank you for reading this tutorial.