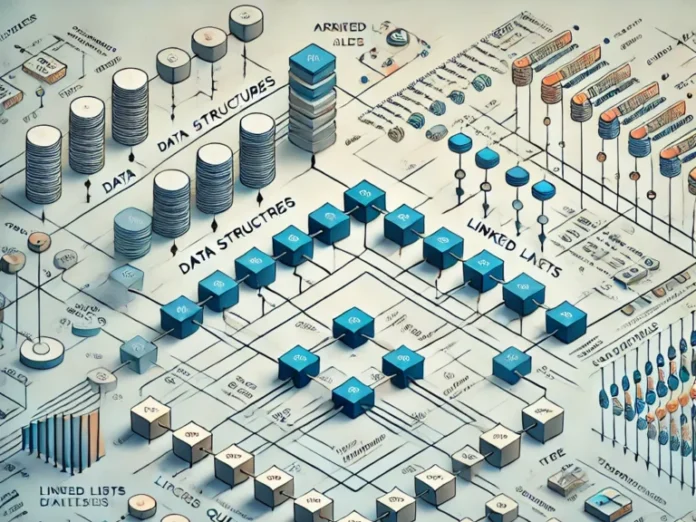
Understanding data structures and algorithms is crucial for every programmer. Whether you are starting with coding or want to improve your problem-solving skills, having a firm grasp on these concepts will set a strong foundation for your career. This guide will walk you through the basics, providing clear examples, and explain why data structures and algorithms are so important for every developer.
Post Contents
What are Data Structures?
Data structures refer to ways of organizing and storing data so that it can be accessed and modified efficiently. They are essential because they make handling large amounts of data easy, allowing developers to write optimized code.
Why Do We Need Data Structures?
To understand why data structures are important, let’s consider a simple scenario. Imagine you are tasked with storing and retrieving a list of students in a class. You could store them in a simple list, but what if you need to frequently search, add, or delete students? Without an efficient structure, this could slow down your application, especially as the list grows.
Types of Data Structures
- Arrays
- Arrays store elements in a contiguous block of memory. They provide fast access to elements using an index.
- Example: Imagine a row of lockers where each locker holds a student’s name. You can quickly open locker number 4 to access the student’s details.
students = ["Alice", "Bob", "Charlie"] print(students[1]) # Outputs: Bob
- Linked Lists
- Linked lists consist of nodes where each node contains data and a reference (link) to the next node.
- Example: Think of it like a treasure hunt, where each clue (node) leads you to the next one.
class Node: def __init__(self, data): self.data = data self.next = None
- Stacks
- Stacks follow the Last In, First Out (LIFO) principle. You can only add or remove elements from the top.
- Example: Imagine a stack of books where you can only take or add the book from the top.
stack = [] stack.append("Book 1") # Add to the stack stack.pop() # Remove from the stack
- Queues
- Queues work on the First In, First Out (FIFO) principle.
- Example: Think of it like a line at the ticket counter—first in line is the first to get served.
from collections import deque queue = deque(["Alice", "Bob", "Charlie"]) queue.append("David") queue.popleft() # Removes "Alice"
- Trees
- Trees consist of nodes with a hierarchical structure, often used for representing relationships or hierarchical data.
- Example: Imagine a family tree where each node represents a family member.
- Hash Tables
- Hash tables store key-value pairs and allow fast lookups. They are efficient for finding, inserting, or deleting data.
- Example: Think of a dictionary where each word (key) has a corresponding definition (value).
What are Algorithms?
An algorithm is a set of instructions or steps designed to solve a problem or perform a task. In programming, algorithms help you process data efficiently. Understanding algorithms enables you to write code that can solve complex problems quickly.
Why Are Algorithms Important?
Algorithms help in solving problems efficiently. Without good algorithms, even the most well-structured data might be slow to process, making your application less responsive.
Types of Algorithms
- Sorting Algorithms
- Sorting algorithms arrange elements in a particular order (ascending or descending). Common algorithms include Bubble Sort, Merge Sort, and Quick Sort.
- Example: Sorting a list of student names alphabetically.
students = ["Charlie", "Alice", "Bob"] sorted_students = sorted(students) print(sorted_students) # Outputs: ['Alice', 'Bob', 'Charlie']
- Search Algorithms
- Search algorithms help locate specific elements within a data structure. The most common search algorithms include Linear Search and Binary Search.
- Example: Searching for a student’s name in a sorted list.
def linear_search(arr, target): for i in range(len(arr)): if arr[i] == target: return i return -1
- Dynamic Programming
- Dynamic programming solves complex problems by breaking them down into simpler overlapping sub-problems.
- Example: Solving a maze by considering smaller sections of the path one at a time.
- Greedy Algorithms
- Greedy algorithms make locally optimal choices at each step, aiming for a global optimum.
- Example: Finding the shortest route in a map by choosing the nearest city at each step.
Data Structures and Algorithms in Real-World Applications
Data structures and algorithms are not just theoretical concepts. They are used in everyday applications that we interact with, such as:
- Search Engines: Google uses sophisticated algorithms to search and rank web pages.
- Social Media Platforms: Facebook and Instagram use algorithms to suggest friends or content.
- E-commerce Websites: Platforms like Amazon use algorithms to recommend products based on your shopping history.
Best Practices for Learning Data Structures and Algorithms
If you’re just starting out, keep these tips in mind:
- Practice Coding Regularly
Hands-on practice is crucial for mastering data structures and algorithms. Solve problems on platforms like LeetCode, HackerRank, or Codeforces. - Understand Time and Space Complexity
Learn how to analyze the efficiency of your algorithms. Focus on the Big O notation, which helps in understanding the time and space complexity of your solution. - Start with Simple Data Structures
Begin with arrays and linked lists before moving on to more complex structures like trees and graphs. - Solve Real-World Problems
Apply what you’ve learned to real-world problems to see how data structures and algorithms play a role in optimizing solutions.
Conclusion
Mastering data structures and algorithms is essential for every developer, regardless of your experience level. They form the backbone of efficient coding, enabling you to tackle complex problems in an organized manner. As you continue your learning journey, remember that practice and application are key to becoming proficient in these areas. With this beginner’s guide, you now have the foundation to dive deeper into more advanced topics in data structures and algorithms.