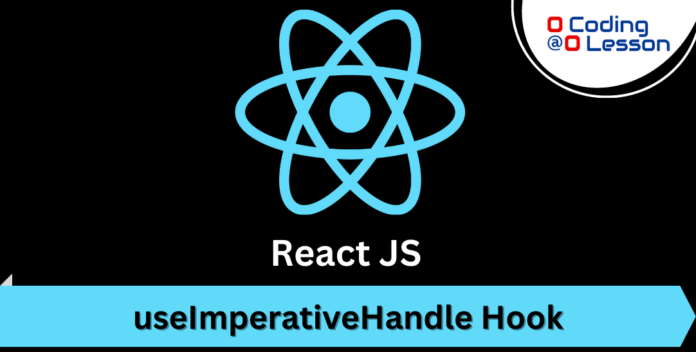
React is a powerful JavaScript library that allows developers to build user interfaces with ease. One of the many features that React useImperativeHandle hook, which allows you to customize the behavior of a child component. In this blog post, we will be discussing the useImperativeHandle hook in detail and show you how to use it in your React applications.
It is a hook in React that allows you to pass a ref to a child component and control its behavior. It is typically used when you need to forward a ref to a child component that is not directly rendered by React. The hook takes two arguments, the first being the ref object, and the second being an object that contains the methods you want to expose to the parent component.
Using useImperativeHandle can be a bit tricky and it’s important to understand when and how to use it properly. It’s best practice to use useImperativeHandle in conjunction with forwardRef to properly pass refs down to child components, and also to have a good understanding of how refs work in React.
In summary, React useImperativeHandle is a hook that enables you to pass refs and methods to child components and control their behavior from the parent component. It’s a powerful feature that can help you optimize your code, but it’s important to use it carefully and understand when it’s appropriate to use it.
Let’s say we have a parent component that renders a child component called “Input”. The parent component wants to have access to the input’s value, so it can use it for other purposes.
import React, { useRef, useImperativeHandle } from 'react';
function Input({ forwardRef }) {
const inputRef = useRef(null);
useImperativeHandle(forwardRef, () => ({
focus: () => {
inputRef.current.focus();
},
getValue: () => {
return inputRef.current.value;
}
}));
return <input ref={inputRef} />;
}
const InputWithRef = React.forwardRef((props, ref) => {
return <Input {...props} forwardRef={ref} />
});
export default function App() {
const inputRef = useRef(null);
const handleClick = () => {
inputRef.current.focus();
console.log(inputRef.current.getValue());
};
return (
<div>
<InputWithRef ref={inputRef} />
<button onClick={handleClick}>Get value</button>
</div>
);
}
In this example, we use the useRef hook to create a ref for the input element in the child component. Then, we use the useImperativeHandle hook to pass the ref and two methods (focus
and getValue
) to the parent component. We use the forwardRef function to pass the ref from the parent component to the child component, and then use the ref in the parent component to call the methods we exposed from the child component.
In this way, the parent component can now focus the input element and retrieve its value by calling the focus() and getValue() methods, respectively.
It’s important to note that the useImperativeHandle hook should be used with caution, and only when it is truly needed. It’s often better to pass down props and use callback functions to communicate between components, rather than using refs.