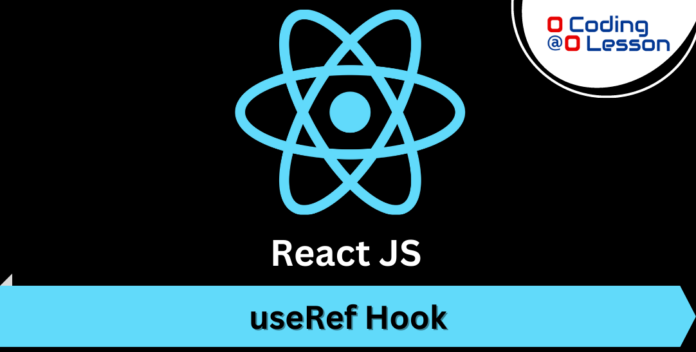
React useRef hook is a powerful tool that allows you to access and manipulate DOM elements in your components. This hook can be used to create references to elements, store values that don’t change with render, and more.
In this comprehensive guide, we’ll walk through the basics of the React useRef hook and explore some of its most common use cases. We’ll also provide examples of how to use the hook in different scenarios and troubleshoot some common issues.
First, let’s start by understanding the basic syntax of the useRef hook. To use the hook, you need to import it from the React library and call it within your component. The hook returns an object with a current property, which you can use to store a reference to an element or a value.
import { useRef } from 'react';
function MyComponent() {
const ref = useRef(initialValue);
return (
<div ref={ref}>
{/* component content */}
</div>
);
}
You can then use the ref.current property to access the referenced element or value. For example, you can use the ref to focus on an input field or set the value of a variable that doesn’t change with render.
function MyComponent() {
const inputRef = useRef();
function handleClick() {
inputRef.current.focus();
}
return (
<div>
<input ref={inputRef} type="text" />
<button onClick={handleClick}>Focus Input</button>
</div>
);
}
In addition to elements, you can use the React useRef hook to store any value that doesn’t change with render. This can be useful for storing timers, intervals, and other state that you need to access outside of the component.
function MyComponent() {
const timerRef = useRef();
useEffect(() => {
timerRef.current = setInterval(() => {
console.log('tick');
}, 1000);
return () => clearInterval(timerRef.current);
}, []);
return (
<div>
<button onClick={() => clearInterval(timerRef.current)}>Stop Timer</button>
</div>
);
}
As you can see, the React useRef hook is a powerful tool that allows you to access and manipulate DOM elements and state in your components. With the knowledge and examples provided in this guide, you should be able to use the hook to improve your own projects.