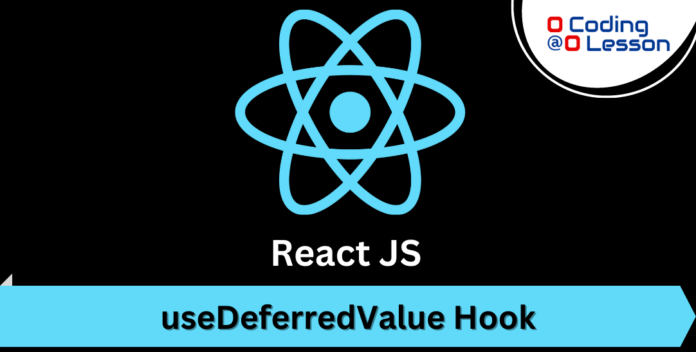
In this blog post, we’ll take a look at the react useDeferredValue hook and how it can be used to improve the performance of your React applications. The useDeferredValue hook allows you to defer the rendering of a component until a certain condition is met. This can be particularly useful when working with large, complex data sets or when trying to optimize the performance of a component that is re-rendered frequently.
To give you a better understanding of how the useDeferredValue hook works, we’ll walk through a real-world example. Let’s say you have a component that displays a list of items. Each item has a title, description, and image. The component is re-rendered every time the user clicks on an item. However, this can cause the component to become slow and unresponsive if the list of items is large.
To solve this problem, we can use the useDeferredValue hook to defer the rendering of the component until the user stops clicking on items. This way, the component is only re-rendered once the user has finished interacting with it, which improves the performance of the component.
Here’s an example of how you can use the useDeferredValue hook to achieve this:
import { useState, useDeferredValue } from 'react';
function ItemList({ items }) {
const [selectedItem, setSelectedItem] = useState(null);
const deferredSelectedItem = useDeferredValue(selectedItem, { timeoutMs: 300 });
return (
<div>
{items.map(item => (
<div
key={item.id}
onClick={() => setSelectedItem(item)}
>
{item.title}
</div>
))}
{deferredSelectedItem && (
<div>
<h2>{deferredSelectedItem.title}</h2>
<p>{deferredSelectedItem.description}</p>
<img src={deferredSelectedItem.image} alt={deferredSelectedItem.title} />
</div>
)}
</div>
);
}
In this example, we’re using the useDeferredValue hook to create a new state variable called deferredSelectedItem
. This variable is set to the value of the selectedItem
state variable, but it is only updated after a delay of 300 milliseconds. This means that if the user clicks on multiple items within 300 milliseconds, the component will only be re-rendered once, after the user has finished clicking on items.