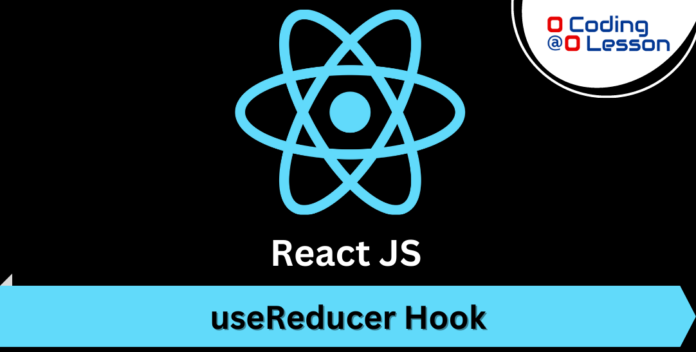
As a React developer, you may have come across a situation where the useState hook becomes unwieldy to manage the state of your application. The useReducer hook is a powerful alternative for managing state in a more organized and efficient way. In this guide, we’ll explore what the useReducer hook is, how it differs from useState and how to use it with examples.
Post Contents
What is the useReducer Hook?
The useReducer hook is a built-in React hook that allows you to manage state by creating a “reducer” function that updates the state based on an action. This approach is similar to that of Redux, a popular state management library for React. The useReducer hook takes two arguments: a reducer function and an initial state.
The reducer function is a pure function that takes in the current state and an action, and returns the new state. The initial state is the starting point for your state management.
const [state, dispatch] = useReducer(reducer, {count: 0});
function reducer(state, action) {
switch (action.type) {
case 'increment':
return {count: state.count + 1};
case 'decrement':
return {count: state.count - 1};
default:
throw new Error();
}
}
In this example, we have a reducer function that takes in the current state and an action, and returns the new state. We use the useReducer hook to create the state variable and update function. The initial state is set to {count: 0}
.
How is useReducer Different from useState?
The useState hook is a simpler way to manage state in React. It allows you to create a state variable and a function to update that variable. However, as your application grows in complexity, useState can become unwieldy. With useState, you may end up with a lot of state variables and update functions, making it difficult to keep track of what’s happening in your application.
The useReducer hook, on the other hand, allows you to manage all of your state in a single place. This makes it easier to understand how your state is changing and what is causing those changes. Additionally, useReducer allows for more complex state updates, such as handling async operations.
Example:
const [user, setUser] = useState({ name: 'John', age: 25 });
function handleClick() {
setUser({ name: 'Mike', age: 30 });
}
In this example, we have a useState hook that manages the state of a user. The state is initialized with the name and age of the user. The setUser function is used to update the state of the user.
How to use React useReducer
Using the useReducer hook is relatively simple. First, you’ll need to create your reducer function. The reducer function takes in the current state and an action and returns the new state.
Next, you’ll use the useReducer hook to create your state variable and update function. You can then use the update function (dispatch) to dispatch actions and update the state.
Finally, you’ll use the state variable to render your component.
Example:
function Counter() {
const [state, dispatch] = useReducer(reducer, { count: 0 });
return (
<>
<h1>{state.count}</h1>
<button onClick={() => dispatch({ type: 'decrement' })}>-</button>
<button onClick={() => dispatch({ type: 'increment' })}>+</button>
</>
);
}
In this example, we have created a simple counter component that uses the useReducer hook. The component has two buttons “+” and “-“, which when clicked, dispatch an action of type ‘increment’ and ‘decrement’ respectively. The reducer function receives the current state and the action and returns the new state based on the action type. The component then renders the current count from the state.
In conclusion, the useReducer hook is a powerful tool for managing state in React. It allows you to manage all of your state in a single place, making it easier to understand how your state is changing and what is causing those changes. Additionally, useReducer allows for more complex state updates, such as handling async operations. The examples provided in this guide should give you a good starting point for using the useReducer hook in your own projects.